Projects
Digit Reader ConvNet
PyTorch, Swift, Objective-C
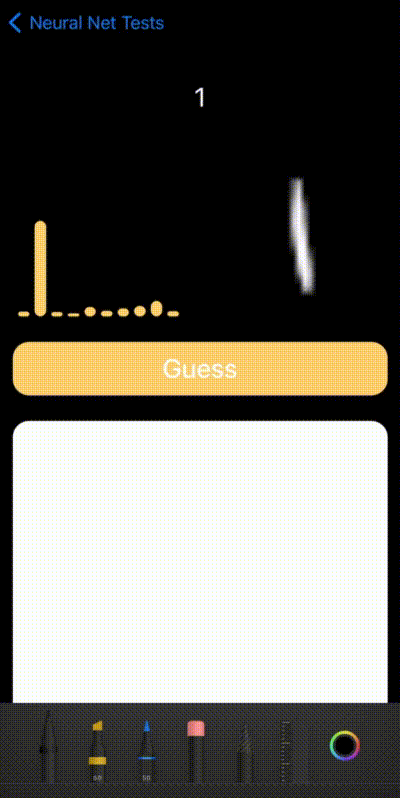
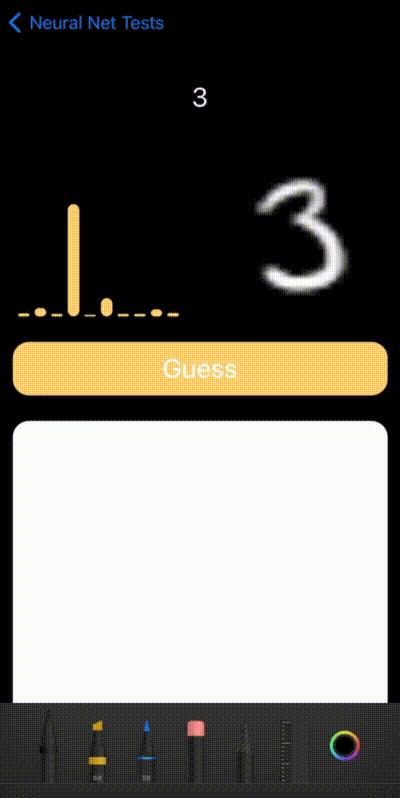
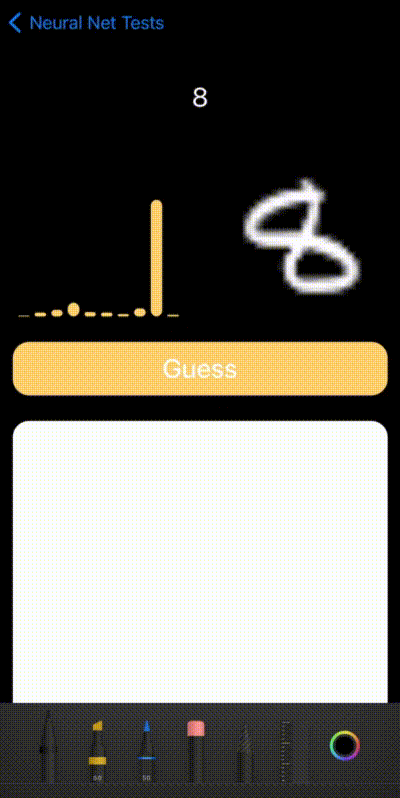
This is my "hello world" machine learning/computer vision project: a simple convolutional neural network trained in PyTorch on the MNIST dataset. I wanted to be able to test the model on my own input, so I built an iOS app where I can draw numbers on the screen and see how well it can infer my drawings.
The network has gone through a couple of iterations in order to get to the above performance. It was relatively easy to achieve high accuracy in a couple of epochs of training with earlier iterations of the architecture, but refinement and normalization to correct overfitting were required for it work acceptably on the real world input.
Below are the final network layers I used. See the source code for hyperparameters - it takes two 2/3 epochs to converge to about 99% accuracy on the MNIST validation set.
(convolutional_layers): Sequential(
(0): Conv2d(1, 32, kernel_size=(5, 5), stride=(1, 1))
(1): ReLU()
(2): MaxPool2d(kernel_size=2, stride=2, padding=0, dilation=1, ceil_mode=False)
(3): Conv2d(32, 64, kernel_size=(5, 5), stride=(1, 1))
(4): ReLU()
(5): MaxPool2d(kernel_size=2, stride=2, padding=0, dilation=1, ceil_mode=False)
)
(linear_layers): Sequential(
(0): Linear(in_features=1024, out_features=1024, bias=True)
(1): ReLU()
(2): Dropout(p=0.2, inplace=False)
(3): Linear(in_features=1024, out_features=10, bias=True)
)
)
Music Streaming App
Angular, TypeScript, Go, MongoDB
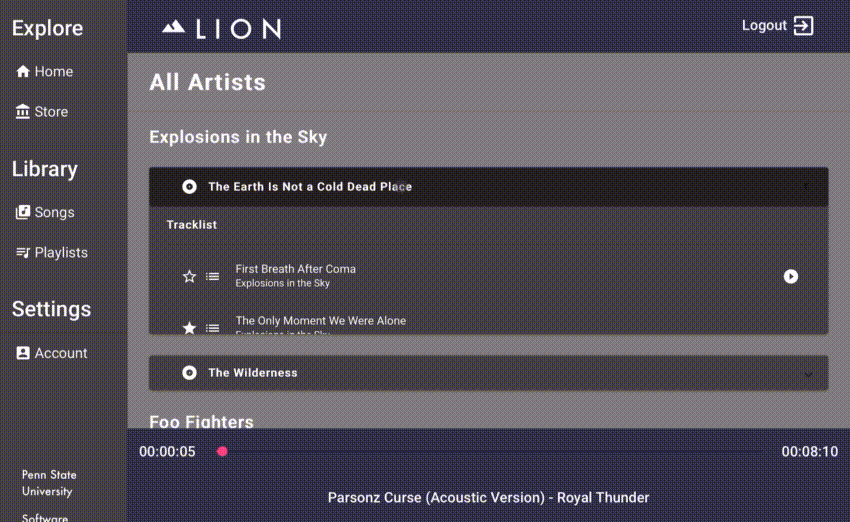
Lion was a music streaming web application that I developed for my Master's Capstone project. The project team consisted of 5 members: a scrum master, two developers, and two on testing/documentation.
I acted as the lead developer/architect for the application, as well as the database admin. The project started with me setting up the all of the endpoints for account management and MP3 file serving/streaming on the backend in sprint 1, completing the core backend functionality; however, frontend development was stalled. I was asked to take over frontend development, and recreated a new application from the ground up, and moved to that version going forward. This allowed me to develop a functional application by the end of sprint 2, and accelerate the feature set development in sprint 3.
Mark Matson.io
Next.js, React, TypeScript
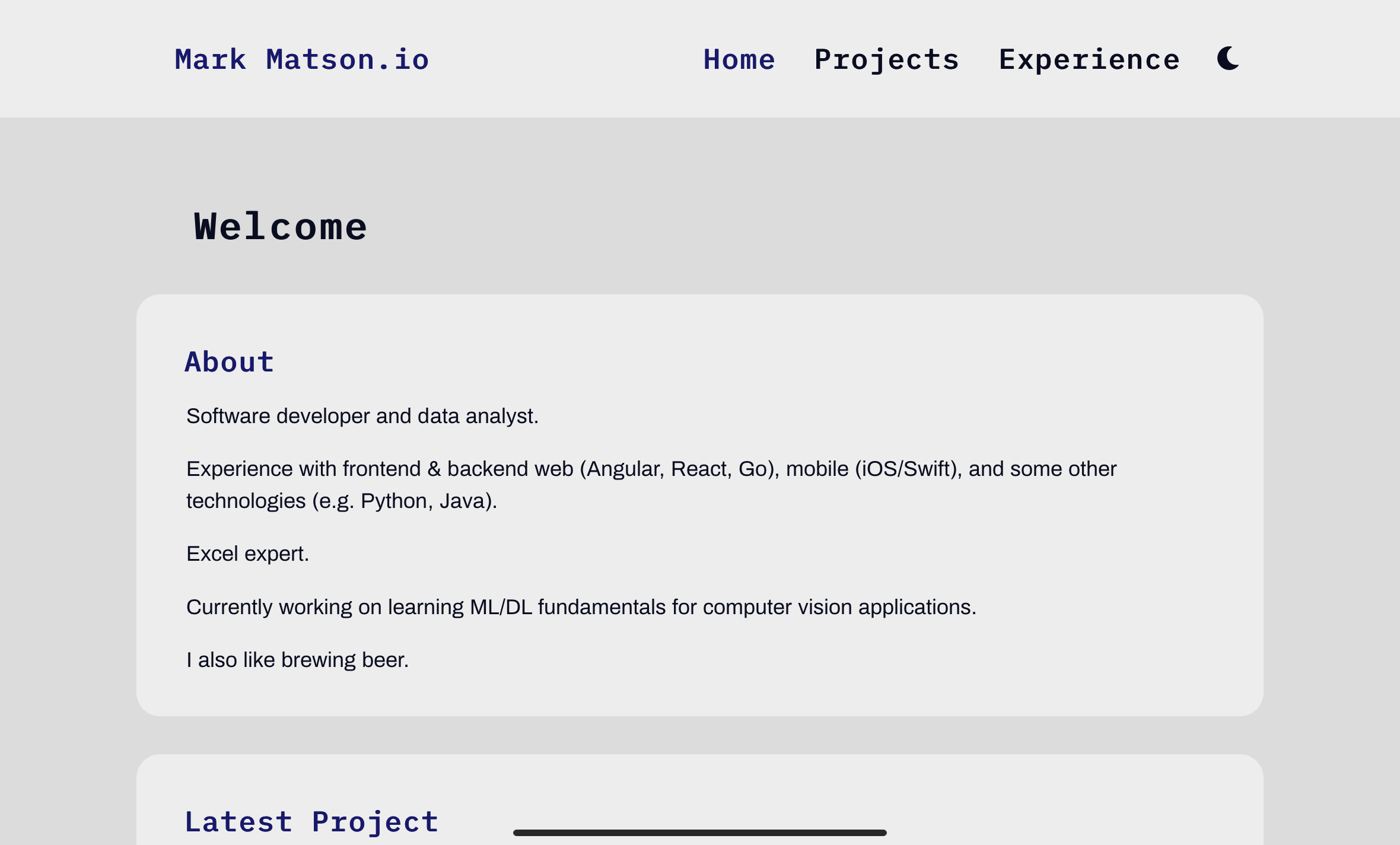
For this site I wanted to get exposure to another TS/JS framework (React). I also wanted to do the CSS, dark mode, etc. myself, which means it's... probably not the best.